Power Panel: My new app to control common computer functions
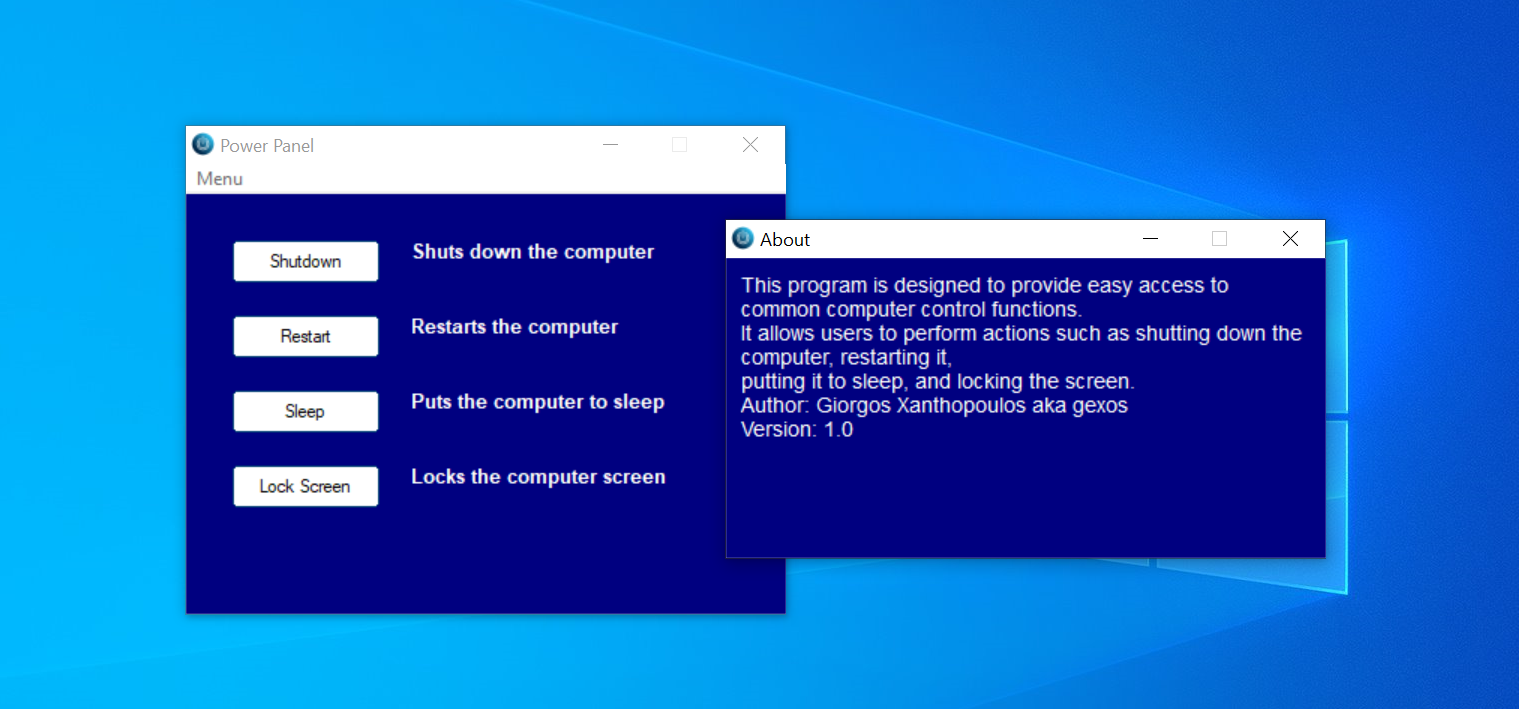
I made this script to automate common computer control functions, simplifying the daily tasks of managing system power and security. With the click of a button or a selection from the menu, users can effortlessly initiate actions such as shutting down or restarting their computer, putting it to sleep, or locking the screen. Whether it's powering down at the end of the day or securing the screen during breaks, this script streamlines the user experience, enhancing productivity and peace of mind.
The Power Panel script provides a user-friendly graphical interface for performing common computer control functions. It offers buttons for shutting down, restarting, putting the computer to sleep, and locking the screen. Additionally, it includes a menu bar for accessing these functions and displaying system information
How It Works
Upon execution, the Power Panel GUI window is displayed, featuring buttons labeled with the respective actions they perform. Users can click on the buttons to trigger the desired actions. Here's a brief rundown of each button's functionality:
- Shutdown: Initiates a system shutdown.
- Restart: Triggers a system restart.
- Sleep: Puts the computer into sleep mode.
- Lock Screen: Locks the computer screen.
Furthermore, the script includes a menu bar with options to access these functions and display system information. Users can select the desired action from the menu, providing flexibility in how they interact with the script.
Implementation Details
The script is written in AutoIt, a scripting language designed for automating the Windows graphical user interface and general scripting. It utilizes AutoIt's built-in functions and libraries to create the graphical interface, handle user interactions, and execute system commands.
For instance, the DllCall
function is used to call Windows API functions responsible for actions like putting the computer to sleep and locking the screen. Additionally, the script leverages AutoIt's GUI functions to create and manage the graphical elements of the interface, such as buttons, labels, and menus.
The actual code:
#include <GUIConstantsEx.au3>
#include <WindowsConstants.au3>
#include <MsgBoxConstants.au3>
; Declare global variables
Global $hGUI, $hMenu, $ID_SHOW_INFO = 1000, $ID_EXIT = 1001, $hInfoWindow
; Create the main GUI window
$hGUI = GUICreate("Power Panel", 400, 300)
GUISetBkColor(0x000080) ; Set background color to dark blue
; Create buttons for different computer control functions
Global $btnShutdown = GUICtrlCreateButton("Shutdown", 30, 30, 100, 30)
GUICtrlSetBkColor($btnShutdown, 0xFFFFFF) ; Set button background color to white
Global $btnRestart = GUICtrlCreateButton("Restart", 30, 80, 100, 30)
GUICtrlSetBkColor($btnRestart, 0xFFFFFF)
Global $btnSleep = GUICtrlCreateButton("Sleep", 30, 130, 100, 30)
GUICtrlSetBkColor($btnSleep, 0xFFFFFF)
Global $btnLock = GUICtrlCreateButton("Lock Screen", 30, 180, 100, 30)
GUICtrlSetBkColor($btnLock, 0xFFFFFF)
; Create labels for button information
Global $infoShutdown = GUICtrlCreateLabel("Shuts down the computer", 150, 30, 200, 30)
GUICtrlSetColor($infoShutdown, 0xFFFFFF) ; Set label text color to white
GUICtrlSetFont($infoShutdown, 10, 800) ; Set font size to 10 and weight to bold
Global $infoRestart = GUICtrlCreateLabel("Restarts the computer", 150, 80, 200, 30)
GUICtrlSetColor($infoRestart, 0xFFFFFF)
GUICtrlSetFont($infoRestart, 10, 800)
Global $infoSleep = GUICtrlCreateLabel("Puts the computer to sleep", 150, 130, 200, 30)
GUICtrlSetColor($infoSleep, 0xFFFFFF)
GUICtrlSetFont($infoSleep, 10, 800)
Global $infoLock = GUICtrlCreateLabel("Locks the computer screen", 150, 180, 200, 30)
GUICtrlSetColor($infoLock, 0xFFFFFF)
GUICtrlSetFont($infoLock, 10, 800)
; Create menu
$hMenu = GUICtrlCreateMenu("Menu")
Global $mnuShowInfo = GUICtrlCreateMenuItem("About", $hMenu, $ID_SHOW_INFO)
Global $mnuShutdown = GUICtrlCreateMenuItem("Shutdown", $hMenu)
Global $mnuRestart = GUICtrlCreateMenuItem("Restart", $hMenu)
Global $mnuSleep = GUICtrlCreateMenuItem("Sleep", $hMenu)
Global $mnuLock = GUICtrlCreateMenuItem("Lock Screen", $hMenu)
Global $mnuSystemInfo = GUICtrlCreateMenuItem("System Info", $hMenu)
GUICtrlCreateMenuItem("", $hMenu, 0)
Global $mnuExit = GUICtrlCreateMenuItem("Exit", $hMenu, $ID_EXIT)
; Show the main GUI window
GUISetState(@SW_SHOW)
While 1
Switch GUIGetMsg()
Case $GUI_EVENT_CLOSE
Exit ; Exit the script when the main GUI window is closed
Case $btnShutdown, $mnuShutdown
Shutdown(1) ; Shutdown the computer when the "Shutdown" button is clicked or menu item is selected
Case $btnRestart, $mnuRestart
Shutdown(2) ; Restart the computer when the "Restart" button is clicked or menu item is selected
Case $btnSleep, $mnuSleep
DllCall("PowrProf.dll", "none", "SetSuspendState", "int", 0, "int", 0, "int", 0) ; Put the computer to sleep
Case $btnLock, $mnuLock
DllCall("user32.dll", "bool", "LockWorkStation") ; Lock the computer screen
Case $mnuSystemInfo
Run("msinfo32.exe") ; Open the System Information utility
Case $mnuShowInfo
ShowInfo() ; Show information about the program when "Show Info" is selected from the menu
Case $mnuExit, $ID_EXIT
Exit ; Exit the script when "Exit" is selected from the menu
EndSwitch
WEnd
; Function to show information about the program
Func ShowInfo()
$hInfoWindow = GUICreate("About", 400, 200)
GUISetBkColor(0x000080) ; Set background color to dark blue
; Text explaining the purpose of the program
$purposeText = "This program is designed to provide easy access to common computer control functions." & @CRLF & _
"It allows users to perform actions such as shutting down the computer, restarting it," & @CRLF & _
"putting it to sleep, and locking the screen." & @CRLF & _
"Author: Giorgos Xanthopoulos aka gexos" & @CRLF & _
"Version: 1.0"
GUICtrlCreateLabel($purposeText, 10, 10, 380, 150)
GUICtrlSetColor(-1, 0xFFFFFF) ; Set label text color to white
GUICtrlSetFont(-1, 11, 400) ; Set font size to 10
; Show the information window
GUISetState(@SW_SHOW, $hInfoWindow)
While 1
Switch GUIGetMsg()
Case $GUI_EVENT_CLOSE
GUIDelete($hInfoWindow) ; Close the information window when the close button is clicked
Return
EndSwitch
WEnd
EndFunc
Explanation of the Code:
#include <GUIConstantsEx.au3>
#include <WindowsConstants.au3>
#include <MsgBoxConstants.au3>
These lines import necessary AutoIt libraries for GUI creation and system constants.
Global $hGUI, $hMenu, $ID_SHOW_INFO = 1000, $ID_EXIT = 1001, $hInfoWindow
Global variables are declared to store handles for the main GUI window, menu, and informational window.
$hGUI = GUICreate("Power Panel", 400, 300)
GUISetBkColor(0x000080) ; Set background color to dark blue
Creates the main GUI window titled "Power Panel" with dimensions 400x300 pixels and sets its background color to dark blue.
Global $btnShutdown = GUICtrlCreateButton("Shutdown", 30, 30, 100, 30)
Creates a button labeled "Shutdown" at position (30,30) with dimensions 100x30 pixels.
Global $infoShutdown = GUICtrlCreateLabel("Shuts down the computer", 150, 30, 200, 30)
Creates a label providing information about the "Shutdown" button's functionality.
$hMenu = GUICtrlCreateMenu("Menu")
Creates a menu bar with the label "Menu."
Global $mnuShowInfo = GUICtrlCreateMenuItem("Show Info", $hMenu, $ID_SHOW_INFO)
Creates a menu item labeled "Show Info" under the "Menu" bar.
Global $mnuExit = GUICtrlCreateMenuItem("Exit", $hMenu, $ID_EXIT)
Creates a menu item labeled "Exit" under the "Menu" bar.
GUISetState(@SW_SHOW)
Displays the main GUI window.
While 1
Switch GUIGetMsg()
Case $GUI_EVENT_CLOSE
Exit ; Exit the script when the main GUI window is closed
Case $btnShutdown
Shutdown(1) ; Shutdown the computer when the "Shutdown" button is clicked
Case $mnuShowInfo
ShowInfo() ; Show information about the program when "Show Info" is selected from the menu
Case $mnuExit
Exit ; Exit the script when "Exit" is selected from the menu
EndSwitch
WEnd
- Enters an infinite loop to handle user interactions with the GUI.
- The
Switch
statement checks for various events, including window closure, button clicks, and menu selections. - When the GUI window is closed (
$GUI_EVENT_CLOSE
), the script exits. - If the "Shutdown" button is clicked, the
Shutdown
function is called to shut down the computer. - If "Show Info" is selected from the menu, the
ShowInfo
function is called to display program information. - If "Exit" is selected from the menu, the script exits.
Func ShowInfo()
$hInfoWindow = GUICreate("Information", 400, 200)
GUISetBkColor(0x000080) ; Set background color to dark blue
; Text explaining the purpose of the program
$purposeText = "This program is designed to provide easy access to common computer control functions." & @CRLF & _
"It allows users to perform actions such as shutting down the computer, restarting it," & @CRLF & _
"putting it to sleep, and locking the screen." & @CRLF & _
"Author: Name" & @CRLF & _
"Version: 1.0"
GUICtrlCreateLabel($purposeText, 10, 10, 380, 150)
GUICtrlSetColor(-1, 0xFFFFFF) ; Set label text color to white
GUICtrlSetFont(-1, 10, 400) ; Set font size to 10
; Show the information window
GUISetState(@SW_SHOW, $hInfoWindow)
While 1
Switch GUIGetMsg()
Case $GUI_EVENT_CLOSE
GUIDelete($hInfoWindow) ; Close the information window when the close button is clicked
Return
EndSwitch
WEnd
EndFunc
- Defines the
ShowInfo
function to display information about the program. - Creates a new GUI window titled "Information" with dimensions 400x200 pixels and a dark blue background.
- Displays text explaining the purpose of the program and includes author and version information.
- Sets the text color to white and the font size to 10 for better readability.
- Enters a loop to handle the close event of the information window. When the close button is clicked, the window is deleted, and the function returns.
Conclusion: The Power Panel script shows the capabilities of AutoIt in automating Windows tasks. By providing a user-friendly interface and streamlined functionality, it simplifies common computer control functions, enhancing user productivity and efficiency.
Additional Resources: